To use the universal remote, simply point your remote control at the IR module and press a button on the remote control. Then press the Arduino button whenever you want to retransmit the code. My example only supports a single code at a time, but can be easily extended to support multiple codes.
The hardware
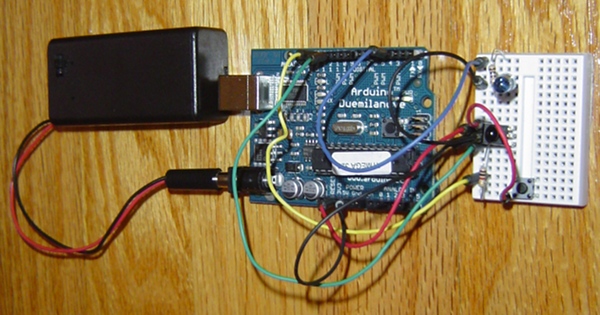
The above picture shows the 9V battery pack, the Arduino board, and the proto board with (top-to-bottom) the IR LED, IR receiver, and pushbutton.
The circuitry is simple: an IR sensor module is connected to pin 11 to record the code, an IR LED is connected to pin 3 to transmit the code, and a control button is connected to pin 12. (My IR library article has details on the sensor and LED if you need them.)
The software
The code can be downloaded as part of my IRremote library download; it is the IRrecord
example.
Handling different protocols
The code supports multiple IR protocols, each with its own slight complications:Sony codes can be recorded and played back directly. The button must be held down long enough to transmit a couple times, as Sony devices typically require more than one transmission.
The common NEC protocol is complicated by its "repeat code". If you hold down a button, the remote transmits the code once followed by multiple transmissions of special repeat code. The universal remote records the code, not the repeat code. On playback, it transmits the code once, followed by the repeat code.
The RC5 and RC6 protocols handle repeated transmissions differently. They use two separate codes for each function, differing in a "toggle bit". The first time you hold down a button, the first code is transmitted repeatedly. The next time you hold down a button, the second code is transmitted repeatedly. Subsequent presses continue to alternate. The universal remote code flips the toggle bit each time it transmits.
The universal remote handles any other unknown protocol as a "raw" sequence of modulated IR on and off. The main complication is that IR sensor modules typically stretch out the length of the "on time" by ~100us, and shorten the "off time" correspondingly. The code compensates for this.
Most likely there are some codes that that can't handle, but it has worked with the remotes I've tried.
The code also prints debugging information to the serial console, which can be helpful for debugging any problems.
In conclusion, this is intended as a proof of concept rather than a useful product. The main limitation is supporting one code at a time, but it's straightforward to extend the code. Also note that the record and playback functions can be separated; if you know the IR codes you're dealing with, you can use just the necessary function.
100 comments:
Very interesting - now if I just had a use for it, it would also be usefull.
I found a use for it, thanks for the library!
http://electrosthetics.blogspot.com/2009/11/arduino-universal-remote-and-more.html
I am using a JVC remote from what I can tell its like a NEC in alot of aspects. But I don't understand where to put in the numbers to decode in IRremote to add JVC as a type.
Thank you for the library. Very easy to use.
I'll be using it to trigger an Olympus camera based on a flying insect.
I can also see a lot of uses in a home automation setup.
The code works very well, however I cannot seem to get it to store and repeat more than one! Do you have any example code for this?
This started to work for me after increasing RAWBUF in IRremote.h to 256. Indeed, 129 bytes were collected from the non-standard remote of my aircondition. Maybe the default buffer is a bit tight here.
To the poster who wonders how to replay what's been received: Copy the received code sequence from the serial terminal, remove the s and m characters and pass the numbers to the sendRaw call.
My proof-of-concept sketch is here: http://pastebin.com/EzNNrYey
Thanks Ken for the great library!
This is one of the easiest thing to check for so you might as well be up front and honest.
I am a noob to Arduino so learning this is a bit difficult so please forgive me. I am trying to control a Tascam DR-100 any ideas where I should start. The DR-100 is controllable by IR and they have a little converter that will make the IR Remote wired but I figured that maybe start with the IR thing then try to get the wired working since it must be the same codes. What I want to do is to have an RTC in arduino start the DR-100 recording at a specific time then stop. There is no sleep in the DR-100 so no need to wake it up. Any clues?
Thanks
I'm doing all this reading and investigation because I've lost the remote for an old receiver & pre-amp and am hoping to get this to work in place of the missing remotes. I've found the proper pronto hex codes on remote-central but i'm still not quite sure how to take the pronto hex info from remote central and get it to be used in the arduino? I guess the part that is confusing to me is that there seems to be so many different modulation frequencies and i'm not sure how or even if that matters? any help would be greatly appreciated.
I am using the Library to control Channel master box....
Here are the commands:
#define cmUP 0x35CA8877
#define cmDOWN 0x35CA08F7
#define cmPOWER 0x35CA38C7
#define cmKEY0 0x35CA00FF
#define cmKEY1 0x35CA807F
#define cmKEY2 0x35CA40BF
#define cmKEY3 0x35CAC03F
#define cmKEY4 0x35CA20DF
#define cmKEY5 0x35CAA05F
#define cmKEY6 0x35CA609F
#define cmKEY7 0x35CAE01F
#define cmKEY8 0x35CA10EF
#define cmKEY9 0x35CA906F
Can someone give me some guidance please?
Am trying to make an Arduino based, dedicated "Closed Caption (CC) toggler".
In order to Toggle the CC, my set-top box's native remote requires eight (yes, eight) keystrokes.
This remote is not RC5. RC6, Sony or NEC.
Have built Arduino send/capture device and have captured RAW data from the set-top box's remote.
Problem:
Have attempted to use "IRrecord" to retransmit codes using Arduino apparatus described in the blog.
CAN do this with RC5 codes, thus demonstrating that hardware works properly.
HOWEVER: not able to do it with the set-top box remote that I actually need to use.
Can anyone direct me to a solution for this problem please?
To summarize:
1. Hardware has been built and tested for RC5
2. Have captured codes from remote, which I wish to retransmit from Arduino driven IR LED.
3. How do I send a sequence of eight sets of RAW to the IR LED and have it be readable by my set-top box.
Thank you for your help with this.
BobW
PS: Have successfully built Ken's Arduino TV-Begone.
BobW: See my comment above from April 9 on how I replayed the received raw codes. Still works for me.
Thank you Martin.
Will get to work on this tomorrow.
BobW
Martin:
I'm up and running thanks to your advice. Thanks so much for your help !
BobW
I have no success with this. Trying to use it with a Creative DDTS-100. I've used values from http://lirc.sourceforge.net/remotes/creative/DDTS-100 and changed IrremoteInt.h like this:
// pulse parameters in usec
#define NEC_HDR_MARK 8973
#define NEC_HDR_SPACE 4760
#define NEC_BIT_MARK 591
#define NEC_ONE_SPACE 1675
#define NEC_ZERO_SPACE 567
#define NEC_RPT_SPACE 2308
I then use IRsendDemo and change the send command:
irsend.sendNEC(0x15EA, 16);
The DDTS doesn't react. I see the IR-beam in my digital camera and have placed the transmitter Led really close to the DDTS.
I used the library to control some costumes in a play. Three characters had LEDs, a 9volt battery,an Ardweeny and a IR receiver. Using a Sony TV remote I was able to send codes to all three costumes simulaneously.
Worked great!
I found the error! Yes!! The answer is in this comment: "Hi reconnnn: I'm in the process of writing an explanation of LIRC data, but for your case LG usually uses 32-bit NEC protocol. Take the pre_data bytes in the LIRC file and join them to the bytes for the button you want to control to make a 4-byte hex number, and use sendNEC. Power on is probably 0x20df10ef; some LG devices use 0x897641be or 0x34347887. If you have an IR detector, you can read out what your remote sends, rather than looking in the LIRC files.
September 26, 2009 3:17 PM " from http://www.arcfn.com/2009/08/multi-protocol-infrared-remote-library.html.
If I use "irsend.sendNEC(0x8322E11E, 32);" the device turns on. Happy, now I can use the DDTS altough the remote went missing.
I've wrote down the process to use a Lirc file with Irremote: http://www.patrikhermansson.se/?q=node/430
Thanks for the great library! Unfortunately IRremote has
side effects on the build-in tone() library. Either sounds are
crippled or - after playing a tone - the receive library stops
working and returns 0 for any IR event.
Is there a way to fix this problem?
Or a workaround?
After a bit of searching and experimenting I stumbled upon this side: http://www.arduino.cc/cgi-bin/yabb2/YaBB.pl?num=1176970454.
So the solution to the tune() imcompatibility is simply this code:
void freqout(int freq, int t)
{
int hperiod; //calculate 1/2 period in us
long cycles, i;
hperiod = (500000 / freq) - 7; // subtract 7 us to make up for digitalWrite overhead - determined empirically
// calculate cycles
cycles = ((long)freq * (long)t) / 1000; // calculate cycles
/* Serial.print(freq);
Serial.print((char)9); // ascii 9 is tab - you have to coerce it to a char to work
Serial.print(hperiod);
Serial.print((char)9);
Serial.println(cycles); */
for (i=0; i<= cycles; i++){ // play note for t ms
digitalWrite(outpin, HIGH);
delayMicroseconds(hperiod);
digitalWrite(outpin, LOW);
delayMicroseconds(hperiod - 1); // - 1 to make up for fractional microsecond in digitaWrite overhead
}
}
It works like charm with IRremote...
I'm trying to run this with a 56KBaud signal (TSOP1156 Receiver) but getting nowhere. As I'm new to Arduino can somebody tell me if I need to change anything anywhere please?
I have no idea of the signal format as it's not a domestic equipment remote that I'm trying to 'grab' and emulate - all I know is that the original equipment uses a TSOP1156 and (according to the info sheet) "The address is encoded into the IR sequence with 5 hamming code bits
and then manchester encoded to give a 32 bit complete code. Since manchester encoded then every transmitter sends 16 high and 16 low pulses. Giving an equal power on the beam. any erroroneous data is ignored by a recevier."
Tham eans absolutely nothing to me bot hopefully it may do to somebody...
Any assistance would be very welcome.
I have this working now (a well known make of prototyping board with a power rail that didn't run the full length!!!) but...
According to my camera phone, the IR LED stays on after the code has been sent - making for short battery life.
Has anyone else noticed this? Anyone have a fix?
It's using RAW, I don't know if that means anything in relation to the problem.
Thanks
Ken, this library is awesome, thanks a bunch. Anyway, I was playing around with sending multiple codes with a single button press. I have one button to turn on the TV and the AV receiver, another button that selects the audio mode on the receiver, etc. This library is so powerful that with macros you can build a serious universal remote rivaling some of the commercial ones available.
I was just stringing commands together with a delay of (40) between each transmission and it seems to work ok. Just wondering if you have a different way of creating macros or if you could recommend optimum delay values, etc.
Thanks for the library Ken. Question: will your examples work with an UNO? I think I have everything right and the codes I recorded match a work lirc configuration but I just don't seem to be able to get the stereo device to recognize the output. Any help is greatly appreciated.
--Chris
I Need a little help with RC5, I was able to transmit using NEC with no problems but my television will not respond to codes using the RC5 protocol.
#include
IRsend irsend;
void setup() {
}
void loop() {
irsend.sendRC5(0x26, 20);
delay(100);
}
what am i doing wrong? what should the number of bits be? 26 is whats reported (in hex) as being the power code.
I am setting up an Arduino Uno to control remote control lights. IRRecord seems perfect to get the data I need. I modified IRRecord to output codelen and to output rawCodes after the irsend.sendRaw command.
Serial output:
Received unknown code, saving as raw
23
m1450 s300 m1250 s400 m450 s1300 m1250 s400 m400 s1300 m400 s1300 m1250 s400 m450 s1250 m400 s1300 m400 s1300 m400 s1250 m400
Pressed, sending
Sent raw
1450 300 1250 400 450 1300 1250 400 400 1300 400 1300 1250 400 450 1250 400 1300 400 1300 400 1250 400
Released
This does not activate the lights. However, if I cut/paste the codes into an array as per Luckylarry's example, it works.
Any idea why IRRecord does not?
Thanks for the library. I am using IRHashdecode for another application with no problems.
Mike
Thanks for the great library!
All remote capture is perfect, but one remote for panasonic tv not recognized.
I try to use panasonic decode function- it do not recognize.
Remote work in Pronto format
dum of recive codes:
1
Raw (76):
-17316 3550 -1650 500 -350 550 -1200 450 -400 550 -350 500 -350 500 -400 450 -400 450 -400 500 -400 500 -350 500 -350 500 -400 450 -400 500 -1250 450 -400 500 -350 500 -400 450 -400 500 -350 500 -400 500 -350 450 -400 500 -400 450 -1300 450 -400 500 -350 500 -400 450 -400 500 -350 500 -400 450 -400 500 -350 500 -400 500 -350 500 -350 550 -350 450
2
Raw (76):
-10870 3450 -1700 500 -350 500 -1250 500 -350 500 -350 500 -400 450 -400 500 -350 450 -450 450 -400 450 -400 500 -350 500 -400 450 -400 450 -1300 450 -400 500 -350 500 -350 450 -450 450 -400 450 -400 500 -400 500 -350 450 -400 500 -1250 500 -350 450 -400 500 -400 450 -400 500 -350 500 -400 450 -400 500 -350 450 -1300 450 -400 500 -350 500 -400 450
3
Raw (76):
-892 3500 -1700 500 -350 500 -1250 450 -400 450 -400 500 -400 450 -400 450 -400 500 -350 500 -400 450 -400 500 -350 450 -450 450 -400 450 -1250 500 -400 450 -400 500 -350 500 -400 500 -350 450 -400 500 -350 550 -350 450 -400 500 -1250 450 -400 450 -400 500 -350 500 -400 450 -400 500 -350 450 -450 450 -400 450 -400 500 -1250 450 -400 500 -350 500
A hint for the new Arduino 1.0 version:
To get this library running under Arduino 1.0 you need to change one line of code in the file IRremoteInt.h:
line 15: from WProgram.h -> Arduino.h
That is all...
Hello, I need help to send a IR command. It is a signal from a IR fan remote. It is 0xC08, 11 bit, but it isn't sony, nec or the other protocol. How can I send it?
Thanks!!
Excuse my bad english, I'm Italian!
At First I was using this, thanks for the guide-lines.
Arduino
Hi,
sorry for my bad english.
I want create a bluetooth remote infrared with Arduino and Android.
I find a java parser for lirc conf file and I want test it.
It is possible create a most generic send function on arduino for codes parsed by Android?
I have all info and data from lirc file and i think it is possible compute a raw send data with Android and send it to arduino via bluetooth. The arduino decode it to IR Led.
First off, awesome library. Works great.
I plan on using multiple infrared sensors. How would the library support that?
I'm looking in the neighborhood of 15-20 infrared sensors.
I Love this project! I am putting this circuit into a pocket watch. Because of the limited space I am using an attiny85. I cannot laod this program onto it without an error however:
IRrecord.cpp: In function ‘void setup()’:
IRrecord.cpp:36:3: error: ‘Serial’ was not declared in this scope
IRrecord.cpp: In function ‘void storeCode(decode_results*)’:
IRrecord.cpp:55:5: error: ‘Serial’ was not declared in this scope
IRrecord.cpp:72:37: error: ‘DEC’ was not declared in this scope
IRrecord.cpp:78:7: error: ‘Serial’ was not declared in this scope
IRrecord.cpp:86:7: error: ‘Serial’ was not declared in this scope
IRrecord.cpp:89:7: error: ‘Serial’ was not declared in this scope
IRrecord.cpp:92:7: error: ‘Serial’ was not declared in this scope
IRrecord.cpp:95:7: error: ‘Serial’ was not declared in this scope
IRrecord.cpp:96:30: error: ‘DEC’ was not declared in this scope
IRrecord.cpp:99:5: error: ‘Serial’ was not declared in this scope
IRrecord.cpp:99:36: error: ‘HEX’ was not declared in this scope
IRrecord.cpp: In function ‘void sendCode(int)’:
IRrecord.cpp:109:7: error: ‘Serial’ was not declared in this scope
IRrecord.cpp:113:7: error: ‘Serial’ was not declared in this scope
IRrecord.cpp:114:33: error: ‘HEX’ was not declared in this scope
IRrecord.cpp:119:5: error: ‘Serial’ was not declared in this scope
IRrecord.cpp:120:31: error: ‘HEX’ was not declared in this scope
IRrecord.cpp:131:7: error: ‘Serial’ was not declared in this scope
IRrecord.cpp:132:33: error: ‘HEX’ was not declared in this scope
IRrecord.cpp:137:7: error: ‘Serial’ was not declared in this scope
IRrecord.cpp:138:33: error: ‘HEX’ was not declared in this scope
IRrecord.cpp:144:5: error: ‘Serial’ was not declared in this scope
IRrecord.cpp: In function ‘void loop()’:
IRrecord.cpp:154:5: error: ‘Serial’ was not declared in this scope
IRrecord.cpp:159:5: error: ‘Serial’ was not declared in this scope
How can I use this sketch with the attiny85?
Hello Ken, i am trying to work with your library in one Arduino MEGA 2560. The sendRaw function is not working. I tested all PWM ports, from 2 to 13 on board, and i could not see the IR Led blinking with my camera. Any ideas ? Thanks a lot!!!!
I just wanted to echo what someone said higher up - the default sending example, using delay(100), does not work with my Sony receiver. Changing it to delay(50) works perfectly. Lower than delay(50) seems to cause issues.
Hi
I have attached a Beolink IR eye (455kHz carrier frequency)
The receiver is picking up a lot of noise when my room is dark and can be canceled by turning on the light.
Also the raw codes I receive with the IRrecvDump is pretty much the same for all the buttons on my remote (a Bang & Olufsen Beo4 remote).
This it what I get when I press the up/down button:
Raw (26): -13900 1650 -1450 1650 -4600 1600 -4600 1650 -4550 1650 -4600 1600 -4600 1600 -4600 1650 -4600 1600 -4600 1600 -4600 1600 -4650 1600 -4650 1600
And I receive the exact same code/pattern for different buttons, but with less/more codes. 22/24/26 raw codes...
Can anyone help my with this?
Thanks!
Hi thanks for the library its great I was just wondering how the IR receive works,is it constantly polling for an interrupt which would then be triggered by the code read in?.
Port this to an android app, whit an IR led plugged in the (mini)USB and you got a winner.
Hi,
i've used the IRrecvDump example and got the following code for my sony tv:
A90
Decoded SONY: A90 (12 bits)
Raw (26): -8278 2400 -600 1200 -600 600 -550 1250 -550 600 -600 1200 -600 600 -600 600 -600 1200 -550 650 -550 600 -600 600 -600 600
A90
Decoded SONY: A90 (12 bits)
Raw (26): -25750 2400 -550 1200 -600 600 -600 1200 -600 600 -550 1250 -550 600 -600 600 -600 1200 -600 600 -550 650 -550 600 -600 600
Now wondering how to play back the IR signal , I already got the IR sender setup properly on my board and trying to under the following codes as from example :
#include
IRsend irsend;
void setup()
{
Serial.begin(9600);
}
void loop() {
if (Serial.read() != -1) {
for (int i = 0; i < 3; i++) {
irsend.sendSony(0xa90, 12); // Sony TV power code
delay(100);
}
}
}
However am not sure what I need to take from the result of IRrecvDump to use in the sending bit , can anybody help please by posting an answer ?
thanks,
javed
Maybe my page can give you some leads? http://patrikhermansson.se/?q=node/430
Try to use a code from Lirc maybe?
Hi, very interesting projects. Is there a limitation to the distance of the IR transmitter LED with respect to the reciever? Thinking of using your project to build a IR controller that will be mounted on the ceiling center of my room and control all the IR devices remotely.
Hi Ken,
I'm trying to decode a NEC code in my board but I receive the following messages:
8F846BAD
Could not decode message
Raw (72): 27436 1100 -600 50 -50 100 -200 50 -250 50 -50 100 -50 100 -50 50 -100 50 -200 100 -200 50 -250 50 -50 100 -200 50 -100 50 -200 100 -200 50 -100 50 -200 100 -50 100 -200 50 -100 50 -50 100 -50 100 -50 50 -100 50 -100 50 -200 100 -50 50 -250 50 -200 100 -200 50 -200 100 -200 100 -4950 1100 -300 50
DF5D6B92
Could not decode message
Raw (72): 18116 1150 -550 50 -100 50 -200 100 -200 50 -100 50 -100 50 -50 100 -50 100 -200 50 -200 100 -200 50 -100 50 -200 100 -50 100 -200 50 -250 50 -50 100 -200 50 -100 50 -200 100 -50 100 -50 50 -100 50 -50 100 -50 100 -50 100 -200 50 -100 50 -200 50 -250 50 -200 100 -200 50 -250 50 -4950 1150 -300 50
942539A0
Could not decode message
Raw (72): 1016 1100 -550 100 -50 50 -250 50 -200 100 -50 50 -100 50 -100 50 -50 100 -200 50 -250 50 -200 100 -50 100 -200 50 -100 50 -200 100 -200 50 -100 50 -200 100 -50 50 -250 50 -50 100 -50 100 -50 50 -100 50 -100 50 -50 100 -200 50 -100 50 -200 100 -200 50 -250 50 -200 100 -200 50 -5000 1100 -300 50
310CFF35
Could not decode message
Raw (72): 11780 1150 -550 50 -100 50 -200 100 -200 50 -100 50 -50 100 -50 100 -50 100 -200 50 -200 100 -200 50 -100 50 -200 100 -50 100 -200 50 -200 100 -50 100 -200 50 -100 50 -50 100 -200 50 -100 50 -100 50 -50 100 -50 100 -50 50 -250 50 -200 100 -50 50 -250 50 -200 100 -200 50 -200 100 -4950 1150 -250 100
BAAC716C
Could not decode message
Raw (72): 9344 1150 -550 100 -50 50 -200 100 -200 50 -100 50 -100 50 -100 50 -50 100 -200 50 -250 50 -200 100 -50 50 -200 100 -50 100 -200 50 -250 50 -50 100 -200 50 -100 50 -200 100 -50 100 -200 50 -100 50 -50 100 -50 100 -50 50 -250 50 -50 100 -200 50 -100 50 -200 100 -200 50 -250 50 -5000 1100 -300 50
DE14404C
Could not decode message
Raw (72): -27698 1150 -550 50 -100 50 -200 100 -200 50 -100 50 -50 100 -50 100 -50 50 -250 50 -200 100 -200 50 -100 50 -200 100 -50 50 -250 50 -200 100 -50 50 -250 50 -50 100 -200 50 -100 50 -200 100 -50 100 -50 100 -50 50 -100 50 -200 100 -50 100 -200 50 -50 100 -200 100 -200 50 -200 100 -4950 1150 -250 100
3F3E464F
Could not decode message
Raw (72): 19416 1100 -600 50 -50 100 -200 100 -200 50 -50 100 -50 100 -50 100 -50 50 -250 50 -200 50 -250 50 -100 50 -200 100 -50 50 -250 50 -200 100 -50 50 -100 50 -200 100 -50 100 -50 50 -250 50 -50 100 -50 100 -50 50 -250 50 -50 100 -200 50 -250 50 -50 100 -200 50 -250 50 -200 100 -4950 1150 -250 100
248C15D9
Could not decode message
Raw (72): 8388 1100 -550 100 -50 100 -200 50 -200 100 -50 100 -50 50 -100 50 -100 50 -200 100 -200 50 -200 100 -50 100 -200 50 -100 50 -200 100 -200 50 -100 50 -50 100 -200 50 -100 50 -100 50 -200 100 -50 50 -100 50 -100 50 -200 100 -50 100 -200 50 -200 100 -50 100 -200 50 -200 100 -200 50 -5000 1100 -300 50
I created a small board with ATmega16U4 to receive a 38Khz code but its not working. Its strange because the code runs fine in the Arduino Leonardo.
I don't know what I'm missing, BTW, I have incremented MARK_EXCESS to 500 but it didn't work.
Regards,
Has anyone tried to record the IR signals for things other than TVs? My Nikon DSLR uses IR for remote control but there is already a library for that, however, I have a small model helicoptor that uses IR instead of RF for control. So yeah... Anyone ever try recordning non TV signals?
Thank you for the great library! I have been wirking on an IR Translator for my entertainment system and have been greatful for all the hints and comments left by others. For others withing to do similar projects remember to use irrecv.enableIRIn(); after you are finnished with irsend as irsend disables irrecv. Also I found it useful to delay the transmission of the translated IR signal. if i did not the devices i was talking to would not respond.
Example code in void loop:
...
if (irrecv.decode(&results)) {
Serial.println(results.value, HEX);
switch (results.value) {
case 0x181803FC:
//do something when power button is pressed
Serial.println("power button pressed");
delay(500);
irsend.sendNEC(0x4B20D32C,32); //Bose power code
delay(500);
irsend.sendNEC(0xC1AA09F6,32); //Projector Power Code
Serial.println("Sent NEC 0xC1AA09F6");
delay(500);
irsend.sendNEC(0xC1AA09F6,32); //Projector Power Code
Serial.println("Sent NEC 0xC1AA09F6");
break;
case 0x18E710EF:
//do something when chanel up pressed
Serial.println("channel up pressed");
break;
...
//after switch use
irrecv.enableIRIn();
Thanks again for all the hard work on this.
Is this library compatible with the arduino due? I have tried to compile and run the example program IRrecvDemo but I have had the following compile error.
C:\Users\Dresden\Desktop\Indicia\Projects\Arduino Due\arduino-1.5.1r2\libraries\IRremote\IRremote.cpp:23: fatal error: avr/interrupt.h: No such file or directory
compilation terminated.
I tried copying the avr directory and pasting it in the IRremote directory, and the specific compilation error went away but now I have a whole lot more errors.
I am not an expert in programming, but it seems like the necessary files are not being located, how do I fix this?
Has anyone decided any Samsung flat screen tvs?
Or know what format they use??? I tried but got the could not decide message
Hi, I am currently trying to change the software on our student laser tag system (running due boards) (who says you can't use violence to teach) to incorporate this brilliant library. So that the kids can also hack old remote controls to use as game components.
I can get it to repsond correctly to The ir codes and transmit. However when you transmit it prevents any further Ir codes being received. I have tried (I think every variation of using irecv.resume() in different locations. Both in the main loop and the subroutines. A snippet of the code is below.
I know it is probably that I can't see the wood for the trees...but does anyone have concrete suggestions as to where the irrecv.resume needs to go.
(ie, can it just go in the second set of subroutines in loop or does it need to be called in every subroutine?, or have I misinterpreted something very basic.)
Regards
John
void loop()
{
if (irrecv.decode(&results)) // have we received an IR signal?
{
translateIR();
for (int z=0; z<2; z++) // ignore 2nd and 3rd signal repeat
{
irrecv.resume(); // receive the next value
}
}
//othercodehere
if(FIRE != 0){ //acts on trigger state
shoot();//performs send function
}
triggers(); // detects trigger state and sets FIRE value
}
estoxeAll sorted, used IR dump demo as the base to solve this. Am now calling the subroutines from within a series of if statements, each with their own resume command.
thanks
Hi.. I was facing a problem that I was not able to playback the IR code using the IRrecord example code. The code was displaying the hexcodes read from the remote control. But it was not replaying the hex code. The circuit used was the same.. except had I used mega2560..I used IRsenddemo also.. but no results..
Please help..
I am having trouble with when I try to load or run the program (IRrecDump)Error is: IRrecv irrecv(RECV_PIN); Also the IRremote.h is not turning color orange in any IR program. I am using Arduino MEGA 2560. Would anyone be so kind and help??
Thank you very much!!
Hi Ken, Thanks a lot for this library. Could you let me know how do I convert the codes received from the remote using IRrecvdump code, into hexadecimal?
Cheers!
I have tried is excatly as decsribed and cannot get it to work. It gets the code, responds to the button, but the IR LED does not shoot. I have troubleshot it in different ways: trying different leds, different resistors different remotes, it won't work. What am I doing wrong???
http://ubiyubix.wordpress.com/2012/05/05/porting-the-arduino-irremote-library-to-the-attiny4313/
how can we use this circuit for multiple functions ?? like for different functions of television by adding more push buttons in the circuit (say 4 functions) ????
Can you please tell me how to change IR library for SAMSUNG LED TV UE40B8000?? Reply me ASAP..Thanks in advance
Can you please tell me how to change IR library for SAMSUNG LED TV UE40B8000?? Reply me ASAP..Thanks in advance
I have use follwing program. but it gives the error like
sketch_jan27a:3: error: 'IRSend' does not name a type
and i incuded IRremote file into library
#include
IRsend irsend
void setup()
{
Serial.begin(9600);
}
void loop()
{
if(Serial.read()!=-1)
{
for(int i=0; i<3; i++)
{
irsend.sendSony(0xa90,12);
delay(40);
}
}
}
I have use follwing program. but it gives the error like
sketch_jan27a:3: error: 'IRSend' does not name a type
and i incuded IRremote file into library
#include
IRsend irsend
void setup()
{
Serial.begin(9600);
}
void loop()
{
if(Serial.read()!=-1)
{
for(int i=0; i<3; i++)
{
irsend.sendSony(0xa90,12);
delay(40);
}
}
}
Hi Ken, thanks a bunch for your work on IR/Arduino, it is amazing and very helpful! I'd like to build a universal remote, that can read in codes from any remote and store them. Do you think the right thing to do is use raw codes or hex codes? I am guessing that if I use hex, then I am at the mercy what I can decode, is that correct?
Hi I've being playing with the irRecord code for a while but I cant figure out on how to do something. Is there some way I can record at least four IR signals and have 4 buttons to send them again
Thank you so much for this marvellous library, which has taught me a lot. I have an 8 gang relay system for controlling different lighting and items in my living room, and as a beginner am getting a bit stuck. I use a universal remote which when pointed at the IR receiver on the arduino board, can successfully receive the eight desired buttons (I have all 10 number keys 0-9 set. My problem is that when I try to modify the program in which a button press toggles on or off the relay, I cannot get it to work with other buttons (1 to 8)
My code is:
#include
int RECV_PIN = 2;
int relay1 = 9;
int relay2 = 10;
int relay3 = 3;
int relay4 = 4;
int relay5 = 5;
int relay6 = 6;
int relay7 = 7;
int relay8 = 8;
IRrecv irrecv(RECV_PIN);
decode_results results;
void setup()
{
pinMode(relay1, OUTPUT);
pinMode(relay2, OUTPUT);
pinMode(relay3, OUTPUT);
pinMode(relay4, OUTPUT);
pinMode(relay5, OUTPUT);
pinMode(relay6, OUTPUT);
pinMode(relay7, OUTPUT);
pinMode(relay8, OUTPUT);
pinMode(13, OUTPUT);
irrecv.enableIRIn(); // Start the receiver
digitalWrite(relay1, HIGH);
digitalWrite(relay2, HIGH);
digitalWrite(relay3, HIGH);
digitalWrite(relay4, HIGH);
digitalWrite(relay5, HIGH);
digitalWrite(relay6, HIGH);
digitalWrite(relay7, HIGH);
digitalWrite(relay8 , HIGH);
}
int on = 0;
unsigned long last = millis();
void loop() {
if (irrecv.decode(&results)) {
if (results.value == 16724175)
if (millis() - last > 250) {
on = !on;
digitalWrite(relay1, on ? HIGH : LOW);
last = millis();
irrecv.resume();
}}}
I tired to add other buttons as the dec codes in between the loop. I copied the HEX codes read from the remote, then converted to DEC via windows calulator. Each code works if I alter the above code to a specific number, but I cannot set it to, for example, Switch on relay 4 then switch off relay 5 then switch oof relay 4 by simply toggle pressing the buttons.Times eight! (though I would be happy to get it to work with four or six. Maybe I have to use arrays in some magical way? Please help, or perhaps point me in the right direction... any thanks, Tyrone
Hi,
After much hair-tugging, I have finally got it all working remote buttons 1 to 8 toggle the 8 gang relay, button 9 switches all on to test and 0 shuts down all eight. :) here's the code if it helps anyone.
#include
int RECV_PIN = 2;
int relay1 = 9;
int relay2 = 10;
int relay3 = 3;
int relay4 = 4;
int relay5 = 5;
int relay6 = 6;
int relay7 = 7;
int relay8 = 8;
IRrecv irrecv(RECV_PIN);
decode_results results;
void setup()
{
pinMode(relay1, OUTPUT);
pinMode(relay2, OUTPUT);
pinMode(relay3, OUTPUT);
pinMode(relay4, OUTPUT);
pinMode(relay5, OUTPUT);
pinMode(relay6, OUTPUT);
pinMode(relay7, OUTPUT);
pinMode(relay8, OUTPUT);
pinMode(13, OUTPUT);
irrecv.enableIRIn(); // Start the receiver
digitalWrite(relay1, HIGH);
digitalWrite(relay2, HIGH);
digitalWrite(relay3, HIGH);
digitalWrite(relay4, HIGH);
digitalWrite(relay5, HIGH);
digitalWrite(relay6, HIGH);
digitalWrite(relay7, HIGH);
digitalWrite(relay8 , HIGH);
}
int on = 0;
unsigned long last = millis();
void loop() {
if (irrecv.decode(&results)) {
if (results.value == 16724175)
if (millis() - last > 250) {
on = !on;
digitalWrite(relay1, on ? HIGH : LOW);
}
if (results.value == 16718055)
if (millis() - last > 250) {
on = !on;
digitalWrite(relay2, on ? HIGH : LOW);
}
if (results.value == 16743045)
if (millis() - last > 250) {
on = !on;
digitalWrite(relay3, on ? HIGH : LOW);
}
if (results.value == 16716015)
if (millis() - last > 250) {
on = !on;
digitalWrite(relay4, on ? HIGH : LOW);
}
if (results.value == 16726215)
if (millis() - last > 250) {
on = !on;
digitalWrite(relay5, on ? HIGH : LOW);
}if (results.value == 16734885)
if (millis() - last > 250) {
on = !on;
digitalWrite(relay6, on ? HIGH : LOW);
}
if (results.value == 16728765)
if (millis() - last > 250) {
on = !on;
digitalWrite(relay7, on ? HIGH : LOW);
}
if (results.value == 16730805)
if (millis() - last > 250) {
on = !on;
digitalWrite(relay8, on ? HIGH : LOW);
}
if (results.value == 16738455)
if (millis() - last > 250) {
on = !on;
digitalWrite(relay1, HIGH);
digitalWrite(relay2, HIGH);
digitalWrite(relay3, HIGH);
digitalWrite(relay4, HIGH);
digitalWrite(relay5, HIGH);
digitalWrite(relay6, HIGH);
digitalWrite(relay7, HIGH);
digitalWrite(relay8 , HIGH);
}
if (results.value == 16732845)
if (millis() - last > 250) {
on = !on;
digitalWrite(relay1, LOW);
digitalWrite(relay2, LOW);
digitalWrite(relay3, LOW);
digitalWrite(relay4, LOW);
digitalWrite(relay5, LOW);
digitalWrite(relay6, LOW);
digitalWrite(relay7, LOW);
digitalWrite(relay8 , LOW);
}
last = millis();
irrecv.resume(); // Receive the next value
}}
Can anyone figure out why my Visio TV will come on with the 0x20DF10EF NEC code as duplicated from two different remotes with one power button, but not turn back off?
Thank you very much for your awesome library! But I have a strange behavior: I use both (Ir receiver and Ir transmitter) on my Arduino development (based on ATMega2560). The reception works fine, but the receiver sometimes doesn't work properly. I notice it's when Ir transmitter receive something, just after, or almost, the transmitter doesn't send something; the next try is fine after. I can't analyse what. But I think this trouble comes from interrupt function in IRremotelib.
Thanks for your help guys!
What is the range of the IR supposed to be for the transmitter IR led? I am only getting about 6 inches.
Is there possible to control two or more IR emitters independently using IRremote library?
Hi, my name is David and I built a remote to control the power, volume, and channels of my TV. While away at school it works but when I got home I hooked it back up to the Comcast Cable box I have and the channels no longer work (because its changing the TV channels, and not the cable channels)(the TV needs to stay on channel 3) Ive been trying to figure out how to get my remote to send out the comcast remote channel codes but can't seem to figure it out. Anyone have any ideas? Id really appreciate it. Thank you!!!
How can I translate Codes from the LIRC Format to your IRSend-Format?
An example would be nice.
hi,can somebody explain to me how to decode signal from air conditioner remote control...i already got some the code 0xB24D7B84 (on) and 0xB24D3FC0 (off)...it detects as samsung protocol but when i try to transmit the signal to my air conditioner irsend.sendSAMSUNG(0xB24D7B84,32)...nothing happen to the air condition..can somebody help me with this topic..or am i wrong somewhere..
I used the library to control Sharp AQUOS TV LC-40LE830E by emulation commands of remote control GA902WJSA. The library recieved RAW data for button POWER ON/OFF as one array with 32 elements (first OFF pulse + 31 main pulses). I sent this array (without first OFF pulse) with sendRaw(), but nothing happend. Then I found this article http://www.ladyada.net/wiki/tutorials/learn/sensors/ir.html and tried recieve RAW data by sketch from this article. And I recieved RAW data array as six series of 31 pulses with some pause between series (~45 msec). Then I sent those six series of 31 pulses one by one with sendRaw() with delay 45 msec. And this works! TV reacts on this command, switches OFF and switches ON.
I analyzed those six series and found that only two are main. So, I declared these series:
unsigned int tvOnOff1[31] = { 320, 1720, 320, 720, 320, 720, 320, 720, 320, 720, 320, 720, 320, 1720, 320, 1720, 320, 720, 320, 1720, 320, 720, 320, 720, 320, 720, 320, 1720, 320, 720, 300 };
unsigned int tvOnOff2[31] = { 320, 1720, 320, 720, 320, 720, 320, 720, 320, 720, 320, 1720, 320, 720, 320, 720, 320, 1720, 320, 720, 320, 1720, 320, 1720, 320, 1720, 320, 720, 320, 1720, 300 };
And wrote code:
irsend.sendRaw(tvOnOff1, 31, 38);
delay(45);
irsend.sendRaw(tvOnOff2, 31, 38);
delay(45);
irsend.sendRaw(tvOnOff1, 31, 38);
delay(45);
irsend.sendRaw(tvOnOff2, 31, 38);
delay(45);
irsend.sendRaw(tvOnOff1, 31, 38);
delay(45);
irsend.sendRaw(tvOnOff2, 31, 38);
Still, thanks to the author for the library! :-)
saiful nizam,
try sketch from this article http://www.ladyada.net/wiki/tutorials/learn/sensors/ir.html for reading RAW data. Then try send RAW data by method sendRaw() of this library. Maybe it will help!
Fandm, how did you get those arrays? I have a Sharp TV and I have been trying to get the raw codes right forever with no luck. But by using the ones you got with the same 45 second interval repeated 6 times worked for me. I also ran the sketch to decode raw from http://www.ladyada.net/wiki/tutorials/learn/sensors/ir.html but the raw data I got from there isn't close to what you got.
I have been using this Library for a few days now. It's been a very frustrating process. I have come to the conclusion your choice of Infra Red Detector is crucial! I can see the pulses appearing but they seem to be incredibly short! This might mean I get a lot of 50uSec pulse widths, then a big gap. I surmise that some IR detectors are much different to others possibly toggling their outputs on negative and positive edges rather than what I am seeing. I can press the same button twice with a pause in between and get a wildly differing output! Has anyone got any suggestions about this?
Hi,
Can I use the same configuration with a lil bit edition in the code to make it learn codes and save it in eeprom? also regenerate the codes to transmit specific code from eeprom? has anyone tried it
Hello
I used this library on MTK LinkIt ONE with Arduino IDE 1.5.7.
When I compiled example code, I got following error message. Could you help me to solve it? Thanks.
D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp: In member function 'void IRrecv::enableIRIn()':
D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:115:6: error: 'cli' was not declared in this scope cli();
In file included from D:\arduino-1.5.7\libraries\IRremote\IRremote.h:24:0, from D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:1:
D:\arduino-1.5.7\libraries\IRremote\IRremoteInt.h:246:3: error: 'TCCR2A' was not declared in this scope
TCCR2A = _BV(WGM21); \
D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:120:2: note: in expansion of macro 'TIMER_CONFIG_NORMAL'
TIMER_CONFIG_NORMAL();
In file included from D:\arduino-.5.7\hardware\arduino\mtk\cores\arduino/Arduino.h:45:0, from D:\arduino-1.5.7\libraries\IRremote\IRremoteInt.h:24, from D:\arduino-1.5.7\libraries\IRremote\IRremote.h:24, from D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:1:
D:\arduino-1.5.7\libraries\IRremote\IRremoteInt.h:246:16: error: 'WGM21' was not declared in this scope
TCCR2A = _BV(WGM21); \
D:\arduino-1.5.7\hardware\arduino\mtk\cores\arduino/wiring_constants.h:250:25: note: in definition of macro '_BV'
#define _BV(bit) (1 << (bit))
D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:120:2: note: in expansion of macro 'TIMER_CONFIG_NORMAL'
TIMER_CONFIG_NORMAL();
In file included from D:\arduino-.5.7\libraries\IRremote\IRremote.h:24:0, from D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:1:
D:\arduino-1.5.7\libraries\IRremote\IRremoteInt.h:247:3: error: 'TCCR2B' was not declared in this scope
TCCR2B = _BV(CS21); \
D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:120:2: note: in expansion of macro 'TIMER_CONFIG_NORMAL'
TIMER_CONFIG_NORMAL();
In file included from D:\arduino-.5.7\hardware\arduino\mtk\cores\arduino/Arduino.h:45:0, from D:\arduino-1.5.7\libraries\IRremote\IRremoteInt.h:24, from D:\arduino-1.5.7\libraries\IRremote\IRremote.h:24, from D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:1:
D:\arduino-1.5.7\libraries\IRremote\IRremoteInt.h:247:16: error: 'CS21' was not declared in this scope
TCCR2B = _BV(CS21); \
Sorry
Because the characters can not exceed 4096, so I post on following comment.
The remaining error messages are as follows.
D:\arduino-.5.7\hardware\arduino\mtk\cores\arduino/wiring_constants.h:250:25: note: in definition of macro '_BV'
#define _BV(bit) (1 << (bit))
D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:120:2: note: in expansion of macro 'TIMER_CONFIG_NORMAL'
TIMER_CONFIG_NORMAL();
In file included from D:\arduino-.5.7\libraries\IRremote\IRremote.h:24:0, from D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:1:
D:\arduino-1.5.7\libraries\IRremote\IRremoteInt.h:248:3: error: 'OCR2A' was not declared in this scope
OCR2A = TIMER_COUNT_TOP / 8; \
D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:120:2: note: in expansion of macro 'TIMER_CONFIG_NORMAL'
TIMER_CONFIG_NORMAL();
D:\arduino-1.5.7\libraries\IRremote\IRremoteInt.h:127:24: warning: integer overflow in expression [-Woverflow]
#define USECPERTICK 50
D:\arduino-1.5.7\libraries\IRremote\IRremoteInt.h:234:38: note: in expansion of macro 'USECPERTICK'
#define TIMER_COUNT_TOP (SYSCLOCK * USECPERTICK / 1000000)
D:\arduino-1.5.7\libraries\IRremote\IRremoteInt.h:248:12: note: in expansion of macro 'TIMER_COUNT_TOP'
OCR2A = TIMER_COUNT_TOP / 8; \
D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:120:2: note: in expansion of macro 'TIMER_CONFIG_NORMAL'
TIMER_CONFIG_NORMAL();
D:\arduino-1.5.7\libraries\IRremote\IRremoteInt.h:249:3: error: 'TCNT2' was not declared in this scope
TCNT2 = 0; \
D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:120:2: note: in expansion of macro 'TIMER_CONFIG_NORMAL'
TIMER_CONFIG_NORMAL();
D:\arduino-1.5.7\libraries\IRremote\IRremoteInt.h:222:30: error: 'TIMSK2' was not declared in this scope
#define TIMER_ENABLE_INTR (TIMSK2 = _BV(OCIE2A))
D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:123:2: note: in expansion of macro 'TIMER_ENABLE_INTR'
TIMER_ENABLE_INTR;
In file included from D:\arduino-.5.7\hardware\arduino\mtk\cores\arduino/Arduino.h:45:0, from D:\arduino-1.5.7\libraries\IRremote\IRremoteInt.h:24, from D:\arduino-1.5.7\libraries\IRremote\IRremote.h:24, from D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:1:
D:\arduino-1.5.7\libraries\IRremote\IRremoteInt.h:222:43: error: 'OCIE2A' was not declared in this scope
#define TIMER_ENABLE_INTR (TIMSK2 = _BV(OCIE2A))
D:\arduino-.5.7\hardware\arduino\mtk\cores\arduino/wiring_constants.h:250:25: note: in definition of macro '_BV'
#define _BV(bit) (1 << (bit))
D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:123:2: note: in expansion of macro 'TIMER_ENABLE_INTR'
TIMER_ENABLE_INTR;
D:\arduino-1.5.7\libraries\IRremote\irRecv.cpp:127:6: error: 'sei' was not declared in this scope
sei(); // enable interrupts
hi i would like to ask about to send raw data
this coding to control the fan by apps, i am already test it, but it doesn't work to control on/off fan
#include
IRsend irsend;
String readString;
int ledPin=12;
unsigned int powerOn[24] ={ 24174,1300,400,1300,400,400,1250,1250,400,1250,400,500,1200,450,1200,500,1200,450,1250,400,1250,1250,400,400};
unsigned int powerOff[24]={ 6454,1300,400,1300,350,500,1200,1250,450,1250,350,500,1200,500,1200,400,1250,400,1250,500,1150,500,1200,1250};
void setup() {
Serial.begin(9600);
pinMode(ledPin, OUTPUT);
}
void loop() {
while (Serial.available()) {
delay(100);
char c = Serial.read();
readString += c;
}
if (readString.length() >0) {
if (readString == "ON" )
{
digitalWrite (ledPin,HIGH);
delay(100);
digitalWrite (ledPin,LOW);
irsend.sendRaw(powerOn,24,50);
}
if (readString == "OFF")
{
digitalWrite (ledPin,HIGH);
delay(100);
digitalWrite (ledPin,LOW);
irsend.sendRaw(powerOff,24,50);
}
readString ="";
}
}
Check dis out... Simple & easy to understand.
http://vaaiibhav.me/how-to-send-ir-signal-with-arduino/
Hi, is it possible to use software PWM librairy to send IR on any of the none PWM pins instead of the fixed pin 3?
Good stuff Ken!
i work on some IR transmission system
I got successfully IR Raw code how to convert My IR raw code to pronto hex code?
Is their any sketch for receive directly pronto code?
Please help me.
I tried a lot to decode IR code for Air conditioner.If you give than i will work on it,my mail id is [email protected]
I did read all aspect parameter of Pronto code.
bom
Is there any way to add Arduino 101 support for this library????
OK here's a challenge!
I'm trying to get the Arduino to transmit a Milestag start game command.
The data protocol is described here:
http://lasertagparts.com/mtformat-2.htm
I am trying to transmit the new game command (byte 1 = 0x83, byte 2 = 0x05)
Can anyone assist please?
I was just looking for something to record one of my TV remotes button presses, because if I don't touch the remote for an hour, it gives me 60 seconds to hit the button or the TV will shut off. Really, though, I was hoping for an Android app (but saw some links, just came here first)
House of Gaming (above post, from July 2, 2018, only about 1.5 years ago, but still newest post I see) - your URL is broken. Hope you got help anyway.
"Choose my identity" below is tricky, because Google Account has a checkbox option to have followups mailed to an email address I haven't had for a decade. Maybe it'll just go to my google email? Or if I use Name/URL, and the URL could be an email address I actually still have? Why do they keep using these "I'm not a robot", when page usage could be made to determine of it was a robot or not, just have to use your own algorithm so the robot writers couldn't just write something to bypass it, but it'd be easy to write. Maybe even if you're smart enough, or think about it enough, you could come up with something open source that robots still couldn't bypass.
Oh.. I just remembered hearing they're using it to teach their AI's, they know you're not a robot already.
But the comment I just left will be posted, if approved, right? There's not "your post is awaiting approval" or anything like that to let me know it actually *got* my post, and didn't just trash it, as some web pages tend to do... I have one on the big G called just seeker. Hopefully they can't scrape that, but moderator could let me know if prev. post was received, and ideally (please) don't post this one, as I was just basically telling you my contact.
Thanks for all the hard work on this. One point to note, many modern 'phone cameras have IR filters now, which means you can't see the IR LED doing its thing! Anything from iPhone 5 onwards seems to be filtered. Easy solution, just knock up the decoder for Arduino! I have mine outputting to a 4 line LCD.
Great ideas to learn by in this post. I just bought a "beginners kit" and the learning curve is mostly in the Manual.
My first project was to setup an auto mute function for TV commercials.
Spent a few hours wondering why my transmit was not operating. All began working when the I noticed your project board had Ir send led plunged into the 4th socket header.
I guess this is pin 3 and also D2 or PD2 but physically connects to pin 4.
I guess the first step is to understand the terminology when learning anything new but I thank you for doing the "hard part" for me. Thank you.
-DJ
Hi, thanks for the library and other posts.
Is there anyone have a code for controlling multiple devices? Such as T.v, A.c, fan ...etc?
Thanks
I would like to turn on my TV and HI-FI system at the same time. Is there any way that TV and Arduino IR would receive IR command from the TV remote at the same time and Arduino IR transmitter would subsequently send an IR command to my HI-FI to power it on and switch to the optical input? Thank you
Hi ,sorry for my poor english. I use an atmega32u4 and my Infrared LED not work on pin3! Any ideea if there is a way to use an atmega32u4 like a remote sender? I successfully receive data from TV remote but i can send nothing. Seems there is another pin for the Infrared LED
I have built a streetplan with infrared controlled robotcars.
For this purpose I need to send more than one IR command at the same time (main road: carry on, side road: stop). The IRremote library however only supports a single IR output.
How can I generate more IR-commands simultaneously on different outputpins?
//An IR LED must be connected to Arduino PWM pin 3.
#include
IRsend irsend;
Ton Reuvekamp
The Netherlands
nice
when I try to compile it says that WProgram does not exists
I am trying to use Ken's library control an led. I want the led to come on when the IR button is pushed and to turn off when the button is released (momentary). The problem is when the buttom is pushed it latches on and stays that way. Below is the code I'm using ... can anyone show me how to modify it so it's momentary?
void loop()
{
if (irrecv.decode(&results)) //updates results
{
Serial.println(results.value);
if(results.value==3476004267) {digitalWrite(19, HIGH); // connected to LED
irrecv.resume(); // Receive the next value
}
}
Post a Comment