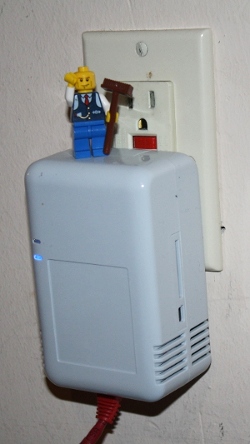
My IPv6 explorations are roughly driven by Hurricane Electric's IPv6 Certification levels. To get from "Newbie" to "Enthusiast" you have to have an IPv6 web server. I expected I could just use the web server you're viewing right now (provided through Pair), but Pair (like most web providers) doesn't support IPv6. So I figured I'd just set up my own simple web server.
To make things more interesting, I decided to set up the server on my Sheevaplug, a very small plug-based computer running Linux. (See my previous articles about Arc on the Sheevaplug, and Arduino with the Sheevaplug.) The things I describe will work just as well on a standard Linux or Windows box, though.
Setting up the SixXS IPv6 tunnel on the Sheevaplug was much easier than setting it up on Windows; I just followed the directions. One gotcha: if your clock is wrong, aiccu will silently fail - check /var/log/syslog.
IPv6 with the Python web server
Python comes with a simple web server. It is easy to configure the web server for IPv6 once you know how, but it took some effort to figure out how to do it.import socket from BaseHTTPServer import HTTPServer from SimpleHTTPServer import SimpleHTTPRequestHandler class MyHandler(SimpleHTTPRequestHandler): def do_GET(self): if self.path == '/ip': self.send_response(200) self.send_header('Content-type', 'text/html') self.end_headers() self.wfile.write('Your IP address is %s' % self.client_address[0]) return else: return SimpleHTTPRequestHandler.do_GET(self) class HTTPServerV6(HTTPServer): address_family = socket.AF_INET6 def main(): server = HTTPServerV6(('::', 80), MyHandler) server.serve_forever() if __name__ == '__main__': main()Most of this code is standard Python SimpleHTTPServer code. It implements a handler for the path
/ip
, which returns the client's IP address. For other paths, SimpleHTTPRequestHandler
returns a file from the current directory; this is not particulary secure, but works for a demonstration.
The key change to support IPv6 is subclassing HTTPServer
and setting the address_family
to IPv6. The other key change is starting the server with the name '::', which is the IPv6 equivalent of '', and binds to no specific address. Similar changes work with related Python classes such as SocketServer.
IPv6 with Arc's web server
My next adventure was to run Arc's web server (which I've documented here) on IPv6. Much to my surprise, Arc's web server worked on Windows with IPv6 without any trouble. You don't have to do anything different to serve on IPv6 and the code and logs handle IPv6 addresses as you'd expect. (Most of the credit for this should go to MzScheme/Racket, which provides the underlying socket implementation.)
I simply start a server thread on port 80, and then define a simple web operation on the home page (represented as ||
for obscure reasons).
arc> (thread (serve 80)) arc> (defop || req (pr "Welcome to Arc! Your IP is " (req 'ip)))Accessing this home page displays a message and the IP address of the client (IPv4 or IPv6 as appropriate).
Unfortunately, when I tried running the same Arc code on the Sheevaplug or another Linux box, it refused to work at all with IPv6. The problem turned out to be misconfiguration in the compilation of Racket (the new name for mzscheme). To get IPv6 working, you can recompile Racket following the instructions here. After recompiling, I was able to get Arc working on my Sheevaplug with IPv6. Eventually this fix will get into the official builds.
Note that implementing web pages in Arc requires much less boilerplate than in Python. This isn't too surprising, given that the primary application of Arc is web serving.
Putting the server's IPv6 address into DNS
Once you have an IPv6 web server, how do you access it? You can access a server with a raw IPv6 address: http://[2001:1938:81:1f8::2]/mypage. (Note that the IPv6 address is enclosed in square brackets, unlike a regular IP address.) However, you'll almost certainly want to access your IPv6 server through a domain name in DNS. To do this, you need to create an AAAA record in your domain zone file.
I had the domain name nlanguages.com available, and wanted to point it at my IPv6 web server. To do this, I went to the DNS Zone File Editor for my nameserver (godaddy.com in my case). I created one AAAA entry for host @ to point to my IPv6 address, and a second AAAA entry for host ipv6 to point to my IPv6 address. The @ provides an entry for my top-level domain (nlanguages.com), and the second provides an entry for ipv6.nlanguages.com. I then tested the DNS entries with a nslookup query, asking for the AAAA record: nslookup -q=aaaa nlanguages.com
The result is that http://ipv6.nlanguages.com will access my IPv6 server (if you have IPv6 access.)
3 comments:
Thanks, this was very useful!
Saved me a lot of pain, thanks!3
Post a Comment